السلام عليكم لدي كود
upload.HTML
<!DOCTYPE html> <html lang="ar"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>رفع الصور والفيديوهات</title> <style> body { font-family: Arial, sans-serif; text-align: center; } #progress-bar { width: 100%; background-color: #f3f3f3; } #progress-bar div { height: 30px; width: 0; background-color: #4caf50; text-align: center; line-height: 30px; color: white; } #result { margin-top: 20px; } </style> </head> <body> <h2>رفع الصور والفيديوهات</h2> <form id="upload-form" enctype="multipart/form-data"> <input type="file" name="file" id="file" accept="image/*,video/*" required> <br><br> <button type="submit">رفع</button> </form> <div id="progress-bar"> <div></div> </div> <div id="result"></div> <script> document.getElementById('upload-form').addEventListener('submit', function(event) { event.preventDefault(); var fileInput = document.getElementById('file'); var file = fileInput.files[0]; var formData = new FormData(); formData.append('file', file); var xhr = new XMLHttpRequest(); xhr.open('POST', 'upload.php', true); xhr.upload.onprogress = function(e) { if (e.lengthComputable) { var percentComplete = (e.loaded / e.total) * 100; document.querySelector('#progress-bar div').style.width = percentComplete + '%'; document.querySelector('#progress-bar div').textContent = Math.round(percentComplete) + '%'; } }; xhr.onload = function() { if (xhr.status == 200) { var response = JSON.parse(xhr.responseText); var resultDiv = document.getElementById('result'); if (response.success) { resultDiv.innerHTML = `<p>تم رفع الملف بنجاح: <a href="${response.url}" target="_blank">${response.url}</a></p>`; resultDiv.innerHTML += `<button onclick="copyToClipboard('${response.url}')">نسخ الرابط</button>`; } else { resultDiv.textContent = 'حدث خطأ أثناء رفع الملف.'; } } else { alert('حدث خطأ أثناء محاولة رفع الملف.'); } }; xhr.send(formData); }); function copyToClipboard(text) { var dummy = document.createElement('input'); document.body.appendChild(dummy); dummy.value = text; dummy.select(); document.execCommand('copy'); document.body.removeChild(dummy); alert('تم نسخ الرابط إلى الحافظة.'); } </script> </body> </html>
و كود upload.php للتعامل مع رفع الصور و الفيديوهات الى قاعدة البيانات
<?php $servername = ""; $username = ""; $password = ""; $dbname = ""; // إنشاء الاتصال $conn = new mysqli($servername, $username, $password, $dbname); // التحقق من الاتصال if ($conn->connect_error) { die("فشل الاتصال: " . $conn->connect_error); } function generateUniqueCode($length = 6) { return substr(str_shuffle('0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'), 0, $length); } $response = array(); if ($_FILES['file']['error'] === UPLOAD_ERR_OK) { $file = $_FILES['file']; $filename = $file['name']; $fileTmpPath = $file['tmp_name']; $fileType = $file['type']; $fileContent = file_get_contents($fileTmpPath); $fileContent = mysqli_real_escape_string($conn, $fileContent); $fileExt = pathinfo($filename, PATHINFO_EXTENSION); $uniqueCode = generateUniqueCode(); while (true) { $codeCheckSql = "SELECT id FROM uploads WHERE unique_code = '$uniqueCode'"; $result = $conn->query($codeCheckSql); if ($result->num_rows == 0) { break; } $uniqueCode = generateUniqueCode(); } $sql = "INSERT INTO uploads (file_name, file_type, file_content, unique_code, file_ext) VALUES ('$filename', '$fileType', '$fileContent', '$uniqueCode', '$fileExt')"; if ($conn->query($sql) === TRUE) { $response['success'] = true; $response['url'] = 'https://ajwa4alearab.freewebhostmost.com/' . $uniqueCode . '.' . $fileExt; } else { $response['success'] = false; $response['error'] = "خطأ في قاعدة البيانات: " . $conn->error; } } else { $response['success'] = false; $response['error'] = "لم يتم رفع الملف بنجاح."; } $conn->close(); header('Content-Type: application/json'); echo json_encode($response); ?>
و كود view.php لعرض الصور و الفيدوهات
<?php $servername = ""; $username = ""; $password = ""; $dbname = ""; // إنشاء الاتصال $conn = new mysqli($servername, $username, $password, $dbname); // التحقق من الاتصال if ($conn->connect_error) { die("فشل الاتصال: " . $conn->connect_error); } if (isset($_GET['code']) && isset($_GET['ext'])) { $code = $conn->real_escape_string($_GET['code']); $ext = $conn->real_escape_string($_GET['ext']); $sql = "SELECT file_name, file_type, file_content FROM uploads WHERE unique_code = '$code' AND file_ext = '$ext'"; $result = $conn->query($sql); if ($result->num_rows > 0) { $row = $result->fetch_assoc(); header("Content-Type: " . $row['file_type']); header("Content-Disposition: inline; filename=\"" . $row['file_name'] . "\""); echo $row['file_content']; } else { echo "الملف غير موجود."; } } else { echo "لم يتم تحديد الملف."; } $conn->close(); ?>
يتم انشاء رابط فريد لكل صورة مثل رابط هذه الصورة
لكن المشكلة ان الصورة تحتاج لاعادة تحميل بشكل مستمر عند وضعها في المقالات او فتحها بشكل مباشر من الرابط اي انها لا يتم تخزينها مؤقتا اي عند كل فتح للصورة يجب اعادة تحميلها لكي تظهر هذه صورة توضيحية
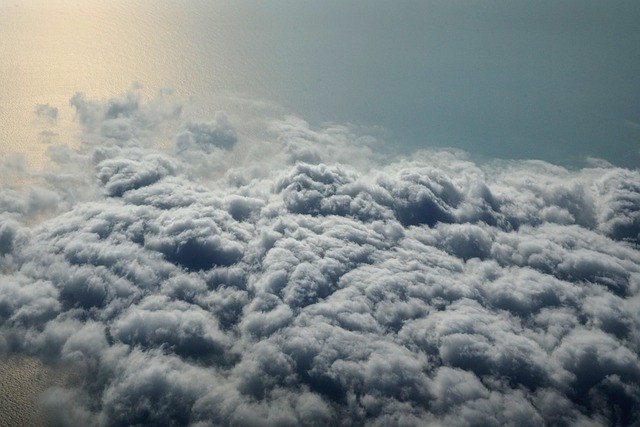
و لكن عند اعادة الدخول يتم اعادة تحميلها من جديد مثل
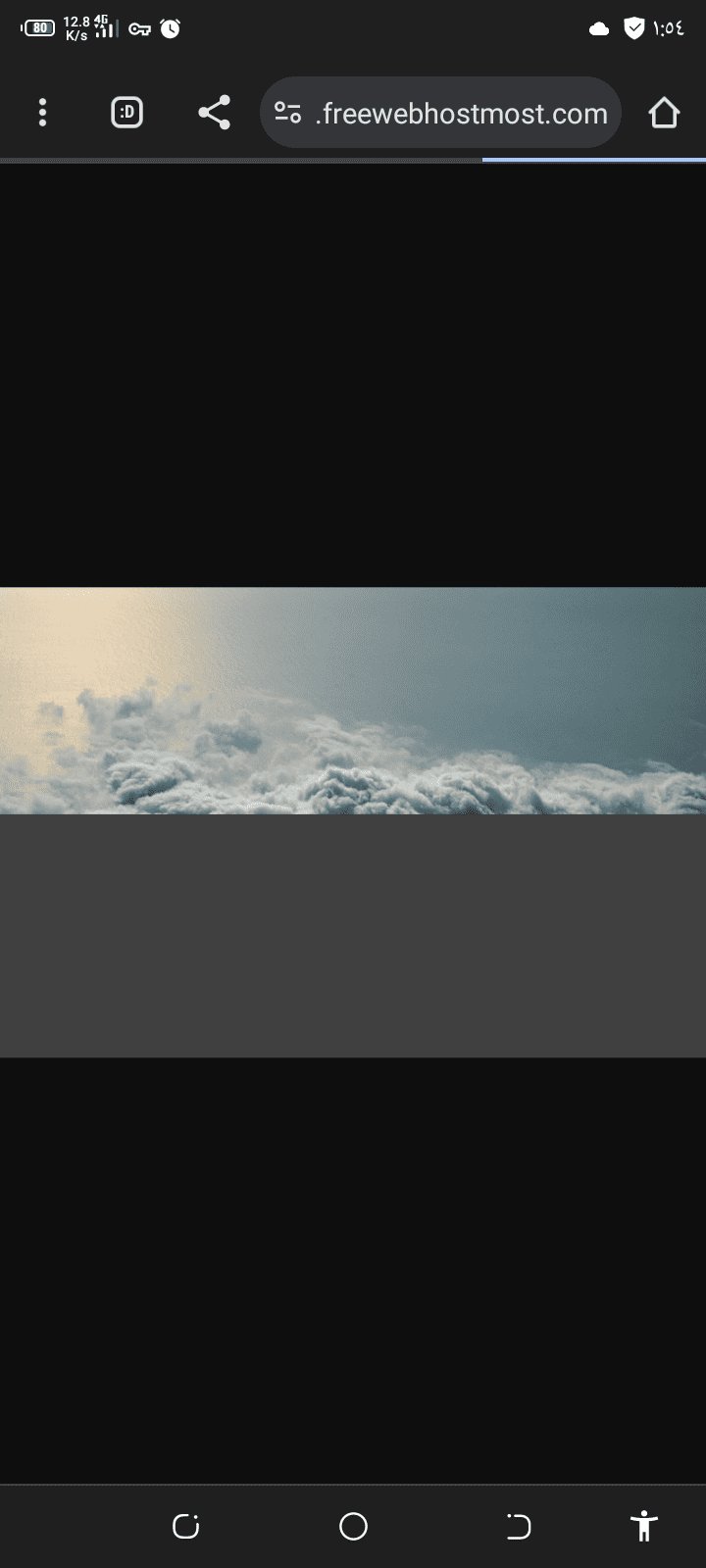
استخدم التالي في ملف htaccess لاعادة توجيه رابط الصورة لصفحة view.php
RewriteEngine On RewriteRule ^([a-zA-Z0-9]{6})\.(jpg|jpeg|png|gif|bmp)$ responsible/view.php?code=$1&ext=$2 [L]
و وضعت التالي ايضا في ملف htaccess لمنع اعادة تحميل الصورة عند كل طلب لكن لا فائدة
<IfModule mod_expires.c> ExpiresActive On ExpiresByType image/jpg "access plus 1 month" ExpiresByType image/jpeg "access plus 1 month" ExpiresByType image/gif "access plus 1 month" ExpiresByType image/png "access plus 1 month" ExpiresByType text/css "access plus 1 month" ExpiresByType application/pdf "access plus 1 month" ExpiresByType text/x-javascript "access plus 1 month" ExpiresByType application/x-shockwave-flash "access plus 1 month" ExpiresByType image/x-icon "access plus 1 month" ExpiresDefault "access plus 2 days" </IfModule>
اعلم اني اطلت كثيرا هذه المرة لكن للضرورة احكام لمن يعرف حل لهذه المشكلة يخبرنا بالحل و شكرا
التعليقات